725. Split Linked List in Parts
1. Question
Given the head
of a singly linked list and an integer k
, split the linked list into k
consecutive linked list parts.
The length of each part should be as equal as possible: no two parts should have a size differing by more than one. This may lead to some parts being null.
The parts should be in the order of occurrence in the input list, and parts occurring earlier should always have a size greater than or equal to parts occurring later.
Return an array of the k
parts.
2. Examples
Example 1:
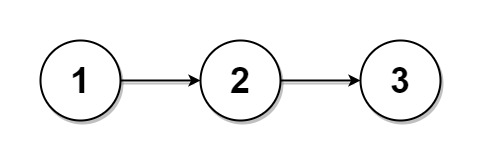
Input: head = [1,2,3], k = 5
Output: [[1],[2],[3],[],[]]
Explanation:
The first element output[0] has output[0].val = 1, output[0].next = null.
The last element output[4] is null, but its string representation as a ListNode is [].
Example 2:

Input: head = [1,2,3,4,5,6,7,8,9,10], k = 3
Output: [[1,2,3,4],[5,6,7],[8,9,10]]
Explanation:
The input has been split into consecutive parts with size difference at most 1, and earlier parts are a larger size than the later parts.
3. Constraints
- The number of nodes in the list is in the range
[0, 1000]
. 0 <= Node.val <= 1000
1 <= k <= 50
4. References
来源:力扣(LeetCode) 链接:https://leetcode-cn.com/problems/split-linked-list-in-parts 著作权归领扣网络所有。商业转载请联系官方授权,非商业转载请注明出处。
5. Solutions
class Solution {
public ListNode[] splitListToParts(ListNode head, int k) {
ListNode node = head;
int count = 0;
while (node != null) {
node = node.next;
count++;
}
// System.out.println(count);
int len = count / k;
// 在第几行之前的所有行数比平均行数多1
int mid = count % k;
ListNode[] nodes = new ListNode[k];
ListNode curr = head;
for (int i = 0; i < k && curr != null; i++) {
int curLen = i < mid ? len + 1 : len;
nodes[i] = curr;
for (int j = 0; j < curLen - 1; j++) {
curr = curr.next;
}
// 备份,断链,恢复赋值
ListNode next = curr.next;
curr.next = null;
curr = next;
}
return nodes;
}
}